In the world of software development and IT operations, DevOps has become the cornerstone of agile, scalable, and reliable software delivery. At the heart of DevOps is automation, and Python, with its simplicity and vast ecosystem, stands out as one of the most powerful tools for this purpose. This article delves into how Python can be effectively used for DevOps, covering key tools, libraries, and real-world applications.
Upgrade Your Skills – Learn Python for Data Science, Automation, and Development.
What is DevOps?
To improve cooperation between software development (Dev) and IT operations (Ops) teams, DevOps combines development and operations methodologies. The objective is to deploy software more quickly without sacrificing security, dependability, or quality.
DevOps Training in Pune provides a great environment to upskills these valuable skills.
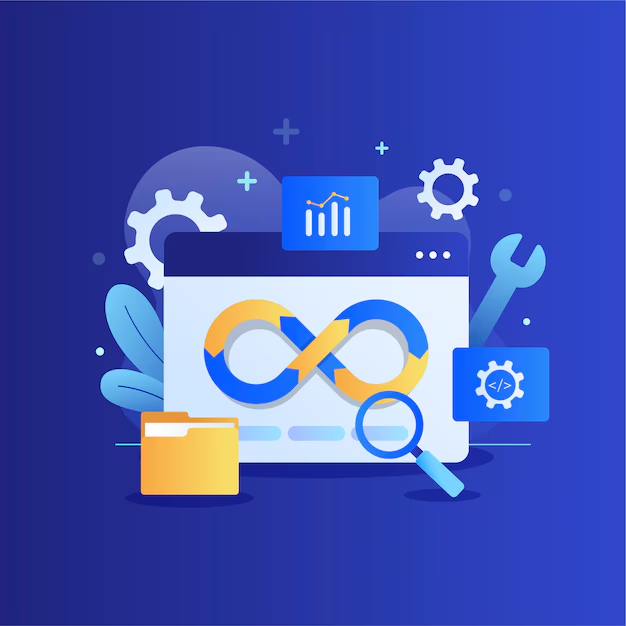
What is Python?
One of the most widely used programming languages worldwide, Python is a high-level, general-purpose language that was developed by Guido van Rossum and initially published in 1991. It is renowned for its readability, simplicity, and adaptability.
Web development, data analysis, artificial intelligence, machine learning, scientific computing, DevOps, and other fields all make extensive use of it. DevOps is more than just a collection of tools or procedures.
It is a cultural movement that prioritizes team communication, integration, and automation.
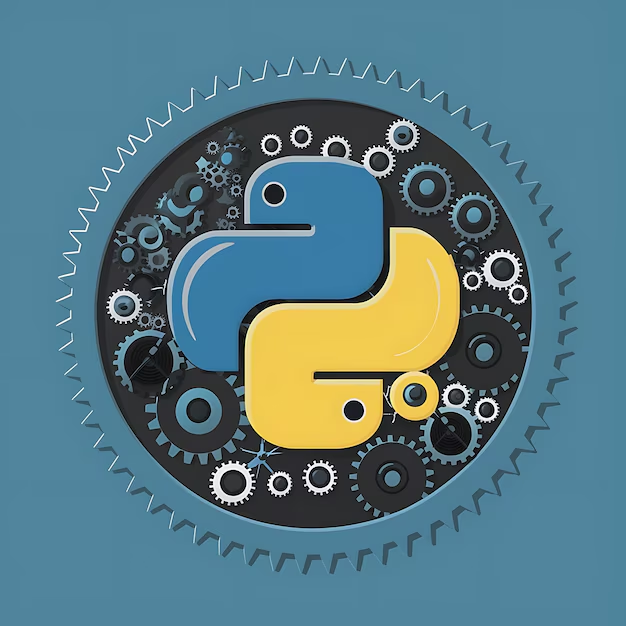
Benefits of Python in DevOps
ChatGPT
Python is a powerhouse in the DevOps world due to its versatility, simplicity, and extensive ecosystem of libraries. Here’s a breakdown of the benefits:
1. Automation
Python excels in writing scripts for automating repetitive tasks like infrastructure provisioning, deployment, configuration management, and monitoring.
Tools like Ansible and Fabric use Python under the hood, enabling seamless task orchestration.
2. Cross-Platform Compatibility
Python works across all major operating systems (Linux, Windows, macOS), which is essential in heterogeneous DevOps environments.
3. Rich Library Ecosystem
Python has libraries for virtually every DevOps requirement:
Paramiko: For SSH connections and remote command execution.
Boto3: For managing AWS services.
Requests: For interacting with APIs.
Pytest: For automating test scripts.
Docker SDK for Python: For container management.
4. Infrastructure as Code (IaC)
Python supports IaC practices through tools like Terraform, Pulumi, and custom scripts, making infrastructure deployment scalable and consistent.
Start Your Coding Journey – Beginner to Advanced Python courses available.
5. Seamless CI/CD Integration
Python scripts can be integrated into CI/CD pipelines for tasks like:
Code linting and formatting.
Automated testing.
Environment setup and cleanup.
Tools like Jenkins, GitLab CI/CD, and CircleCI often use Python for custom scripts.
6. Monitoring and Logging
Python’s libraries (e.g., psutil, loguru, Prometheus client) can help monitor application performance and manage logs efficiently.
7. Collaboration and Community
Python’s simplicity makes it easier for cross-functional teams to understand and contribute to scripts, fostering better collaboration.
A large and active community ensures quick support and access to open-source tools.
8. Scalability
Python can handle both simple one-off scripts and complex, large-scale DevOps tools, making it flexible for teams of all sizes.
9. Cloud and Containerization Support
Python is widely used in cloud environments and container orchestration:
Manage cloud resources via SDKs (AWS, Azure, GCP).
Automate Kubernetes clusters using libraries like Kubernetes Python Client.
10. Learning Curve
Python’s clean syntax and readability make it beginner-friendly, allowing DevOps engineers to adopt it quickly without steep learning curves.
Would you like an example of how Python is used for a specific DevOps task?
How to Use Python for DevOps
1. Why Python for DevOps?
Python is an excellent choice for DevOps due to its:
- Ease of Learning: Python’s intuitive syntax makes it easy for both developers and system administrators to pick up.
- Versatility: From web development to scripting, Python’s use cases span a broad spectrum.
- Rich Ecosystem: Python provides a large number of libraries and frameworks for DevOps tasks.
- Cross-Platform Compatibility: Python scripts can run flawlessly across multiple operating systems.
These qualities make Python the go-to language for automating tasks, managing infrastructure, and streamlining CI/CD pipelines.
2. Automating Tasks with Python
One of the fundamental uses of Python in DevOps is task automation. Python scripts may save time and minimize human error by taking the place of tedious manual activities.
- File Management: Python’s built-in libraries like os and Shutil can handle file operations such as creation, deletion, or organization.
- Log Analysis: With libraries like re for regular expressions and pandas for data manipulation, Python can parse and analyze log files to identify errors or performance issues.
- Scheduled Jobs: Python scripts can be scheduled using tools like cron (on Linux) or libraries like Schedule and APScheduler.
Example:
import os
from date time import datetime
# Cleanup old log files
def clean_logs(log_dir, days):
now = datetime.now()
for file in os.listdir(log_dir):
file_path = os.path.join(log_dir, file)
if os.path.isfile(file_path):
file_age = (now – datetime.fromtimestamp(os.path.getmtime(file_path))).days
if file_age > days:
os.remove(file_path)
print(f”Deleted: {file_path}”)
clean_logs(‘/var/log/myapp’, 30)
3. Infrastructure as Code (IaC) with Python
One essential DevOps technique is Infrastructure as Code (IaC), which enables teams to specify and control infrastructure using code. Python libraries like boto3, Ansible, and Terraform integrations enable IaC practices.
- AWS Automation: Using boto3, Python can manage AWS resources programmatically, from launching EC2 instances to setting up S3 buckets.
Example:
import boto3
# Create an S3 bucket
def create_s3_bucket(bucket_name):
s3 = boto3.client(‘s3’)
s3.create_bucket(Bucket=bucket_name)
print(f”Bucket {bucket_name} created successfully”)
create_s3_bucket(‘my-new-bucket’)
- Ansible and Python: Ansible’s Python API allows for executing playbooks programmatically.
- Terraform Integration: Python’s subprocess module can be used to invoke Terraform commands and manage state files.
4. Continuous Integration and Deployment (CI/CD) Pipelines
Python is instrumental in setting up and managing CI/CD pipelines. Python scripts are supported for bespoke build, test, and deployment procedures by tools such as Jenkins, GitLab CI, and CircleCI.
- Testing Automation: Python testing frameworks such as pytest are capable of automating end-to-end, integration, and unit tests.
- Deployment Scripts: Python scripts can handle application deployment tasks, such as updating configurations, restarting services, and verifying deployments.
Example:
import subprocess
# Deploy application
def deploy_app():
subprocess.run([“git”, “pull”], check=True)
subprocess.run([“docker-compose”, “up”, “-d”], check=True)
print(“Application deployed successfully”)
deploy_app()
5. Monitoring and Alerting
For high availability to be maintained, warnings and system monitoring are essential. Python’s flexibility enables the creation of custom monitoring tools and integration with existing solutions like Prometheus, Nagios, and Datadog.
- Custom Monitoring Scripts: Python libraries such as psutil can monitor system performance (CPU, memory, disk usage) and requests can check application health.
- Alerting: Libraries like smtplib or services like Slack and Twilio APIs can send alerts when anomalies are detected.
Example:
import psutil
import smtplib
# Monitor CPU usage and send alert
def monitor_cpu(threshold):
cpu_usage = psutil.cpu_percent(interval=1)
if cpu_usage > threshold:
send_alert(f”High CPU usage detected: {cpu_usage}%”)
def send_alert(message):
with smtplib.SMTP(‘smtp.example.com’, 587) as server:
server.starttls()
server.login(‘[email protected]’, ‘password’)
server.sendmail(‘[email protected]’, ‘[email protected]’, message)
monitor_cpu(80)
6. Containerization and Orchestration
Working with containers and orchestration tools like Docker and Kubernetes is made easier with Python.
- Docker Automation: Using the docker library, Python can manage containers, images, and networks.
Example:
import docker
# List running containers
def list_containers():
client = docker.from_env()
for container in client.containers.list():
print(container.name, container.status)
list_containers()
- Kubernetes Management: The kubernetes Python client provides APIs to interact with Kubernetes clusters, enabling tasks like pod scaling and resource monitoring.
7. Security and Compliance
Python helps enforce security and compliance in DevOps workflows:
- Static Code Analysis: Tools like bandit and pylint can analyze code for vulnerabilities.
- Secrets Management: Libraries like python-dotenv and integrations with HashiCorp Vault help manage secrets securely.
- Compliance Audits: Python scripts can validate configurations against security benchmarks.
8. Popular Python Libraries for DevOps
Here’s a quick overview of essential Python libraries for DevOps:
- Fabric: Remote server automation and deployment.
- Pytest: Testing frameworks for unit and integration tests.
- Boto3: AWS SDK for Python.
- Docker SDK: Manage Docker containers.
- Kubernetes Python Client: Work with Kubernetes APIs.
- Psutil: System monitoring and resource management.
9. Best Practices for Using Python in DevOps
- Use Virtual Environments: Manage dependencies using venv or pipenv.
- Write Modular Code: Break down scripts into reusable modules.
- Follow PEP 8: Adhere to Python’s style guide for clean and readable code.
- Use CI/CD for Scripts: Test and deploy Python scripts using CI/CD pipelines.
- Log Everything: Use libraries like logging to capture detailed logs for debugging.
Master Python from the basics to advanced concepts with our expert-led courses in Pune.
Conclusion
Whether you are a seasoned DevOps professional or just starting out, Python is a skill worth mastering. Python has become an indispensable tool for DevOps engineers, enabling automation, infrastructure management, CI/CD, monitoring, and security. Its versatility and robust ecosystem make it an ideal choice for tackling DevOps challenges. Teams can streamline their workflows, improve system reliability, and accelerate software delivery by effectively utilizing Python.